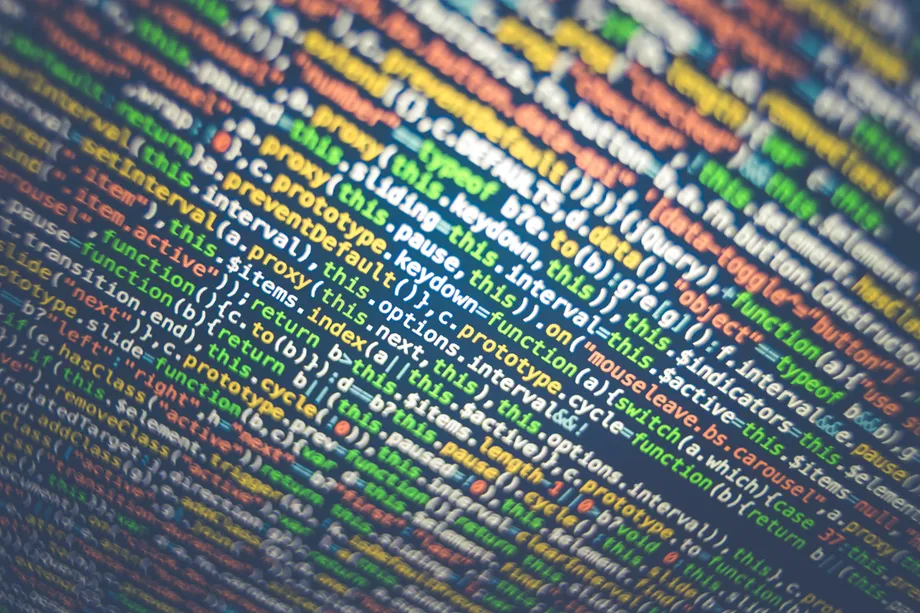
- Harsh Maur
- April 5, 2022
- 04 Mins read
- Scraping
Unlocking the Power of JavaScript and Node.js
Introduction
Web scraping is a potent tool to extract valuable information from the web, enabling businesses and individuals to make data-driven decisions. JavaScript, coupled with Node.js, offers a robust environment to implement advanced web scraping techniques, allowing interaction with dynamic content and automation of browser tasks. This comprehensive guide will explore the advanced methodologies, libraries, and code examples to enhance your web scraping skills and knowledge.
Understanding JavaScript and Node.js in Web Scraping
JavaScript is a versatile programming language, allowing manipulation of the DOM, interaction with web elements, and handling of events. Node.js is a JavaScript runtime built on Chrome’s V8 JavaScript engine, enabling the execution of JavaScript code server-side, making it ideal for web scraping.
Setting Up the Environment
Before diving into advanced techniques, it’s crucial to set up the Node.js environment. Follow the steps below to install Node.js and npm (Node Package Manager):
- Download and install Node.js from Node.js Official Website.
- Verify the installation by running the following commands in your terminal:
node -v npm -v
Puppeteer: A Powerful Library for Browser Automation
Puppeteer is a Node library that provides a high-level API to control headless browsers or full browsers over the DevTools Protocol, making it a go-to choice for web scraping dynamic content.
Installing Puppeteer
Run the following command in your project directory to install Puppeteer:
npm install puppeteer
Basic Usage of Puppeteer
Here’s a simple example of using Puppeteer to open a webpage and take a screenshot:
const puppeteer = require("puppeteer");
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto("https://example.com");
await page.screenshot({ path: "example.png" });
await browser.close();
})();
Advanced Web Scraping Techniques with Puppeteer
-
Navigating to Pages and Extracting Dynamic Content:
-
Example:
const puppeteer = require("puppeteer"); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://example.com"); const dynamicContent = await page.evaluate(() => { return document.querySelector("#dynamic-content").textContent; }); console.log(dynamicContent); await browser.close(); })();
-
Use Case: Extracting content loaded dynamically with JavaScript, such as comments or posts on social media platforms.
-
-
Automating Form Submission and Handling Redirects:
-
Example:
const puppeteer = require("puppeteer"); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://example.com/login"); await page.type("#username", "your_username"); await page.type("#password", "your_password"); await page.click("#submit-button"); await page.waitForNavigation({ waitUntil: "load" }); console.log("Redirected to", page.url()); await browser.close(); })();
-
Use Case: Logging into websites and navigating to different pages to scrape protected content.
-
-
Interacting with Web Elements and Extracting Attributes:
-
Example:
const puppeteer = require("puppeteer"); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://example.com"); const links = await page.$$eval("a", (anchors) => anchors.map((anchor) => anchor.href), ); console.log(links); await browser.close(); })();
-
Use Case: Extracting links or other attributes from multiple elements on a webpage, such as product URLs from a catalog page.
-
-
Handling AJAX Requests and Infinite Scrolling:
-
Example:
const puppeteer = require("puppeteer"); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://example.com"); await page.waitForSelector("#load-more-button", { visible: true }); await page.click("#load-more-button"); await page.waitForTimeout(3000); // Wait for AJAX content to load const additionalContent = await page.$eval( "#additional-content", (el) => el.textContent, ); console.log(additionalContent); await browser.close(); })();
-
Use Case: Loading and extracting additional content on webpages with infinite scrolling or “Load More” buttons.
-
Handling Captchas and Anti-Scraping Mechanisms
Web scraping often encounters challenges such as captchas and anti-bot mechanisms. Here are some strategies to overcome them:
- Using Proxy Servers: Rotating IP addresses using proxy servers can help bypass IP-based blocking.
- Setting Custom User Agents: Changing the user agent can help avoid detection by pretending to be a regular browser.
- Implementing Delays: Introducing delays between requests can mimic human behavior and avoid rate-limiting.
Handling Cookies and Sessions
Managing cookies and sessions is crucial when dealing with websites that require login or have session-specific data.
-
Example:
const puppeteer = require("puppeteer"); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://example.com/login"); // Log in and navigate to the desired page await page.type("#username", "your_username"); await page.type("#password", "your_password"); await page.click("#submit-button"); await page.waitForNavigation({ waitUntil: "load" }); // Extract cookies after login const cookies = await page.cookies(); console.log("Cookies:", cookies); await browser.close(); })();
-
Use Case: Maintaining sessions while scraping multiple pages of a website, especially when dealing with authenticated content.
Web Socket Communication
WebSockets provide a persistent connection between a client and a server, allowing for real-time data transfer. Understanding WebSockets can be crucial for scraping real-time data like chat messages or live sports scores.
-
Example:
const WebSocket = require("ws"); const ws = new WebSocket("wss://example.com/socket"); ws.on("open", function open() { ws.send("Hello Server!"); }); ws.on("message", function incoming(data) { console.log(`Received: ${data}`); });
-
Use Case: Scraping real-time data from websites that use WebSockets to update content dynamically.
Advanced Data Processing and Transformation:
After extracting data, advanced processing and transformation techniques can be applied to clean and format the data according to specific requirements.
-
Example:
const data = [ { name: "John", age: 30, city: "New York" }, { name: "Marie", age: 22, city: "Boston" }, ]; const transformedData = data.map((item) => ({ ...item, fullName: `${item.name} Doe`, ageInTenYears: item.age + 10, })); console.log(transformedData);
-
Use Case: Cleaning and transforming scraped data before storing it, such as converting date formats, concatenating strings, or calculating new values.
Scraping Strategies for Large-Scale Projects
When dealing with large-scale scraping projects, implementing efficient and optimized scraping strategies is crucial to manage resources and time effectively.
-
Example:
const puppeteer = require("puppeteer"); const urls = ["https://example.com/page1", "https://example.com/page2"]; // List of URLs to scrape (async () => { const browser = await puppeteer.launch(); const results = []; for (const url of urls) { const page = await browser.newPage(); await page.goto(url); const result = await page.evaluate(() => { // Extract specific data from each page return document.querySelector("#data-element").textContent; }); results.push(result); await page.close(); } console.log(results); await browser.close(); })();
-
Use Case: Efficiently managing multiple browser pages and resources in large-scale scraping projects, such as scraping data from thousands of URLs.
Error Handling and Recovery
Implementing robust error handling and recovery mechanisms is essential to ensure the scraping process can continue or recover from unexpected failures.
-
Example:
const puppeteer = require("puppeteer"); (async () => { try { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto("https://example.com"); const data = await page.evaluate(() => { // Attempt to extract data return document.querySelector("#non-existent-element").textContent; }); console.log(data); await browser.close(); } catch (error) { console.error("An error occurred:", error.message); } })();
-
Use Case: Gracefully handling errors during the scraping process, such as missing elements or failed navigation, and logging them for analysis.
Optimizing Performance and Resource Management
Optimizing the performance of your scraping code and managing resources effectively is crucial, especially when dealing with large volumes of data or concurrent scraping tasks.
-
Example:
const puppeteer = require("puppeteer"); (async () => { const browser = await puppeteer.launch({ headless: true, args: ["--no-sandbox", "--disable-setuid-sandbox"], }); const [page1, page2] = await Promise.all([ browser.newPage(), browser.newPage(), ]); await Promise.all([ page1.goto("https://example.com"), page2.goto("https://example2.com"), ]); // Perform scraping tasks concurrently on both pages const [data1, data2] = await Promise.all([ page1.evaluate(() => document.querySelector("#element").textContent), page2.evaluate(() => document.querySelector("#element").textContent), ]); console.log(data1, data2); await browser.close(); })();
-
Use Case: Running multiple scraping tasks concurrently to optimize performance and reduce the overall scraping time.
Conclusion
Advanced web scraping techniques using JavaScript and Node.js unlock the potential to interact with and extract data from dynamic and complex webpages. By mastering libraries like Puppeteer and implementing sophisticated strategies to handle challenges, you can elevate your web scraping skills to new heights.