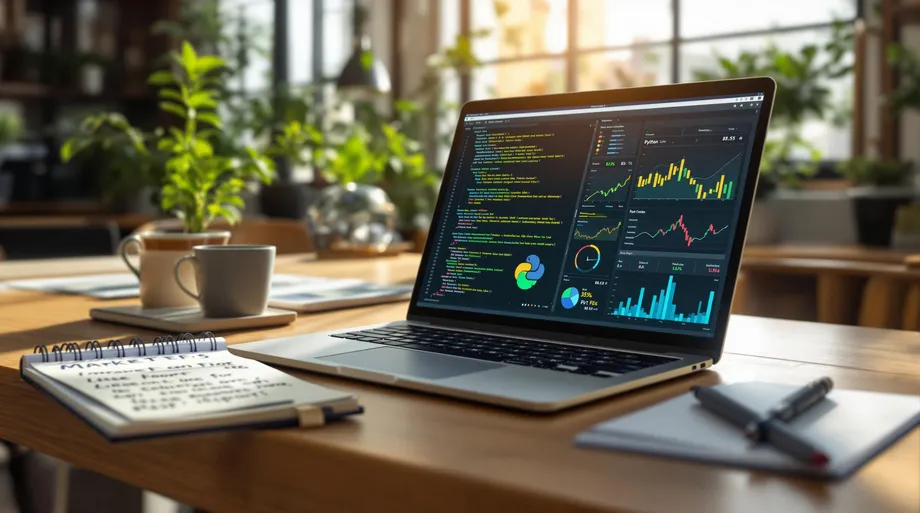
- Harsh Maur
- March 3, 2025
- 10 Mins read
- WebScraping
Explore Business opportunities with Python web scraping
Web scraping is the process of extracting data from websites to gain insights or automate processes. Python is the preferred language for this due to its easy-to-use libraries like BeautifulSoup, Scrapy, and Selenium.
Why Use Web Scraping for Business?
Web scraping helps businesses across industries by:
- Tracking Competitor Prices: Retailers can monitor competitors' pricing in real time.
- Market Analysis: Finance teams can gather alternative data for better investment decisions.
- Lead Generation: Marketing teams can collect contact details for campaigns.
- Trend Monitoring: Companies can analyze social media and review platforms for consumer sentiment.
Getting Started with Python Web Scraping
-
Install Python and libraries like
requests
,beautifulsoup4
, andscrapy
. - Use tools like VS Code or Jupyter Notebook for development.
- Follow ethical practices: check website policies, avoid overloading servers, and comply with data privacy laws.
Advanced Tips for Scaling
- Use rotating IPs and proxies to avoid detection.
- Handle dynamic websites with tools like Selenium.
- Scale operations with distributed systems like Scrapy-Redis.
Quick Comparison of Python Web Scraping Tools
Tool | Best For | Key Features |
---|---|---|
BeautifulSoup | Simple HTML parsing | Easy to use for beginners |
Scrapy | Large-scale scraping | Built-in crawling and scaling |
Selenium | Dynamic content scraping | Handles JavaScript-heavy websites |
Key Takeaway
Python web scraping is a powerful way to gather actionable data for business growth. Whether you're tracking prices, analyzing trends, or generating leads, Python tools make it accessible and efficient.
Getting Started with Python Web Scraping
Required Software Setup
To begin, download the latest stable version of Python from python.org (currently 3.11.7 as of March 2024). Then, install essential libraries using pip by running this command in your terminal:
pip install requests beautifulsoup4 scrapy selenium
These libraries are the core tools for web scraping. BeautifulSoup is widely used for parsing HTML content (with over 10.6 million weekly downloads!), while Requests manages HTTP interactions with websites.
Choosing Development Tools
Your development environment plays a key role in how efficiently you can work on web scraping projects. Here are some commonly used tools:
IDE | Best For | Key Features |
---|---|---|
VS Code | Production scraping | Built-in Git, extensive extensions |
PyCharm | Large-scale projects | Advanced debugging, code analysis |
Jupyter | Learning & testing | Interactive cells, immediate feedback |
For beginners, Jupyter Notebook is a great choice for experimenting and prototyping. On the other hand, professionals often lean toward Visual Studio Code or PyCharm for more complex workflows.
Once your tools are set up, it's important to understand and follow the rules and guidelines surrounding web scraping.
Web Scraping Rules and Ethics
Before diving into scraping, make sure you're aware of the legal and ethical boundaries:
-
Check Website Policies
Always review the site's Terms of Service and robots.txt file. These often outline the site's stance on automated data collection. If no crawl rate is specified, a general rule is to send one request every 10–15 seconds to avoid overloading the server. -
Set a Clear Bot Identity
Use a user agent string to identify your bot clearly. Here's an example:headers = { 'User-Agent': 'Your Company Name Bot 1.0 (contact@yourcompany.com)' }
-
Data Privacy Compliance
Adhere to regulations like GDPR or CCPA when handling data. Public data scraping is typically allowed, but personal information must be managed with strict compliance.
Lastly, consider whether the website offers an official API. For tasks like tracking product prices, APIs are often more dependable and legally straightforward than scraping.
Web Scraping for Business Growth
Web scraping is a powerful tool that helps businesses make informed, data-driven decisions. Companies across various sectors use Python-powered scraping methods to track markets, analyze prices, and gather quality leads.
Tracking Market Trends
Using web scraping for market intelligence allows businesses to stay ahead of changes in their industry. By keeping tabs on competitors, social media platforms, and online marketplaces, companies can spot new patterns and tweak their strategies accordingly.
For example, Amazon uses automated tools to monitor competitor prices, stock levels, and demand trends. These systems process millions of data points, enabling real-time price adjustments to maximize revenue.
Smaller businesses can also benefit by using Python scripts to track specific metrics:
Metric Type | Data Source | Business Impact |
---|---|---|
Product Updates | Competitor websites | Spot new product launches |
Consumer Sentiment | Review platforms | Inform product improvements |
Regional Trends | Google Trends | Focus marketing efforts |
Seasonal Patterns | E-commerce sites | Improve inventory planning |
Keeping an eye on market trends is essential, but competitive pricing is equally important for business growth.
Price Analysis Tools
Python-based price monitoring tools make it easier to stay competitive. These systems perform key tasks such as:
- Collecting pricing data
- Standardizing the data
- Sending alerts when prices change
- Visualizing pricing trends
The airline industry uses similar tools to analyze route pricing. By combining web scraping with machine learning, airlines can predict peak booking periods and adjust prices to boost revenue while staying competitive.
Sales Lead Collection
Web scraping isn't just about trends and pricing - it can also help businesses gather valuable sales leads. For instance, a proptech company expanded its reach nationwide by scraping property directories and feeding the collected contact data into their CRM system.
For effective lead scraping, follow these steps:
- Focus on high-value data sources
- Verify data accuracy
- Use rate limiting to avoid overloading servers
- Clean and remove duplicate contacts
Netflix provides a great example of how data-driven strategies can lead to growth. They analyze user engagement metrics through scraping systems to refine subscription plans. By identifying which features attract and retain users, Netflix has optimized pricing tiers, leading to better customer retention and profitability.
Creating a Basic Web Scraper
Building a web scraper involves careful planning and the right tools. Here’s a step-by-step guide to help you create a scraper tailored to your needs.
Website Analysis
Start by analyzing the structure of the website you want to scrape. Open your browser's developer tools (press F12 in most browsers) to inspect the HTML elements. Look for the specific tags and attributes that contain the data you need. Pay attention to how the page is laid out and check if it uses dynamic content loading (e.g., JavaScript).
Next, review the website's robots.txt file, usually located at domain.com/robots.txt
. This file tells you which parts of the site are open for scraping. Make sure your target pages don't violate these guidelines.
Here’s a quick overview of tools you might need:
Component | Tool | Purpose |
---|---|---|
HTML Parsing | Beautiful Soup | Extract data from static web pages |
JavaScript Handling | Selenium | Manage dynamically loaded content |
Network Requests | Requests | Fetch web pages |
Rate Control | Time/Random | Avoid overloading the web server |
Once you understand the website's structure, you’re ready to start coding.
Data Collection Code
Write code that fetches and parses the required data. For static websites, use the Requests library to fetch pages and Beautiful Soup to extract the desired elements. If the site uses JavaScript to load content, tools like Selenium or Playwright can help you interact with the page and extract data.
Set up proper request headers to mimic a regular browser and avoid detection. Once your basic scraper is working, you can enhance it to handle more complex tasks, like extracting data from multiple pages.
Multi-page Data Extraction
To scrape multiple pages, modify your scraper to navigate through pagination or follow links. Add random delays between requests using time.sleep(random.randint(1, 5))
to avoid triggering anti-scraping measures. Also, make sure your scraper can handle errors gracefully - this prevents crashes and ensures data is saved incrementally.
For websites with dynamic content, check the browser's Network tab to find API endpoints. These endpoints often provide a more efficient way to access the data directly, bypassing the need to scrape rendered HTML.
To stay under the radar, implement techniques like proxy rotation, session management, and random delays. These strategies help reduce the chances of your scraper being detected or blocked.
sbb-itb-65bdb53
Growing Your Web Scraping Business
Taking your basic scraper to the next level can turn raw data into meaningful business opportunities.
Large-Scale Data Collection
Expanding web scraping operations requires distributed systems and cloud-based resources. Tools like Scrapy-Redis can help distribute tasks across multiple servers, enabling load balancing, asynchronous processing, and automated monitoring.
Here’s how to scale effectively:
- Spread tasks across multiple machines.
- Use asynchronous processing to handle multiple requests at once.
- Set up automated monitoring to track performance.
- Build error-handling protocols to minimize disruptions.
"Scrapy-Redis is a powerful open source Scrapy extension that enables you to run distributed crawls/scrapes across multiple servers and scale up your data processing pipelines."
Organizing Your Data
Efficiently managing raw data is critical. For example, in March 2023, Spotify used Mailchimp's Email Verification API to cut its email bounce rate from 12.3% to 2.1% in just 60 days. This change drove a $2.3M revenue boost (Mailchimp Case Studies, 2023).
Phase | Actions | Tools |
---|---|---|
Collection | Remove duplicates | MongoDB |
Cleaning | Validate data accuracy | Python pandas |
Storage | Follow 3-2-1 backup rule | Cloud storage |
Distribution | Deliver in usable formats | JSON/CSV exports |
Organized data doesn’t just improve decision-making - it also creates new ways to generate revenue.
Earning Through Web Scraping
Web scraping can unlock multiple income streams, with service packages often priced between $449 and $999 monthly.
"Cloud browser rendering simulates real user interactions, enhancing efficiency and reducing detection."
Ways to monetize include:
- Building custom APIs tailored to specific websites.
- Selling specialized industry datasets.
- Offering Data as a Service (DaaS) solutions.
- Developing automated monitoring systems.
These approaches can help you stay ahead of the competition while expanding your business.
Advanced Web Scraping Tips
Want to get the most out of your Python web scraping projects? These advanced strategies can help you collect data efficiently while staying within ethical boundaries.
Website Access Rules
Respecting a website’s access rules is crucial. Follow guidelines like those in robots.txt files, introduce random delays, and manage sessions carefully to avoid detection. Tools like ScraperAPI, boasting a 97% success rate, show how proper planning pays off.
Here are some key practices to maintain access:
- Add random delays between requests to mimic normal browsing behavior.
- Stick to site rate limits to avoid overloading servers.
- Regularly check robots.txt for updates to access policies.
- Manage cookies to ensure uninterrupted sessions.
Taking it a step further, using a variety of IP addresses can make your scraping efforts even harder to detect.
Using Multiple IP Addresses
Rotating IP addresses is a smart way to collect data without raising red flags. For example, ScraperAPI’s pool of over 5 million residential IPs demonstrates how large-scale scraping can operate effectively.
Here’s how to rotate IPs effectively:
- Geographic Distribution: Use IPs from different regions to access location-specific data and simulate real-world traffic patterns.
- Proxy Selection: Invest in high-quality proxies for better reliability and avoid free proxies, which are more likely to get blocked.
- Rotation Frequency: Adjust how often you rotate IPs based on the website’s behavior to maintain realistic browsing activity.
These methods help you navigate even the most secure websites.
Bypassing Security Measures
Many modern websites use advanced protections like CAPTCHAs. To avoid triggering these defenses, you’ll need to employ some clever tactics:
- Browser Fingerprinting: Tools like Selenium Stealth can mask automation traits to make your scraper less detectable.
- User Agent Rotation: Regularly switch user agents in headers to mimic different browsers and devices.
- Cookie Management: Keep cookies active to avoid repeated authentication requests.
For example, travel aggregators successfully use these techniques to monitor real-time prices. They rely on advanced IP rotation, headless browser setups, and solid session management to bypass security systems effectively.
Conclusion
Summary of Key Points
Python web scraping plays a major role in driving business growth, contributing to an industry worth $40 billion in 2021. It allows businesses to extract useful data, offering competitive and market insights that support informed decision-making across various sectors.
Here’s what matters most for success:
- Follow website rules and respect data privacy regulations.
- Use reliable proxy and IP rotation systems.
- Ensure data collection and storage are well-organized.
- Develop systems that can grow and perform efficiently.
Take Databoutique.com as an example: they scrape 2 billion prices every month with a small team, proving how impactful web scraping can be. These strategies can help you kickstart your web scraping journey.
Steps to Get Started
Here’s how to dive into web scraping:
-
Lay the Groundwork
Start by learning Python libraries like BeautifulSoup and Scrapy. Focus on markets with strong growth potential, such as sports data analysis, which is expected to hit $3.4 billion by 2028. -
Choose Revenue Streams
Professionals in this field can earn up to $131,500 annually. Popular income sources include building custom solutions, selling data, creating research reports, and running comparison platforms. -
Expand Operations
Look to industry leaders like SEMRush, which began with web scraping tools and grew to a $3.42 billion market cap. Prioritize consistency and high-quality data as you scale.
"Data is the new oil - Clive Humby"
The success of companies like Cars24.com, valued at $1.84 billion in June 2021, highlights how web scraping can unlock new business opportunities.