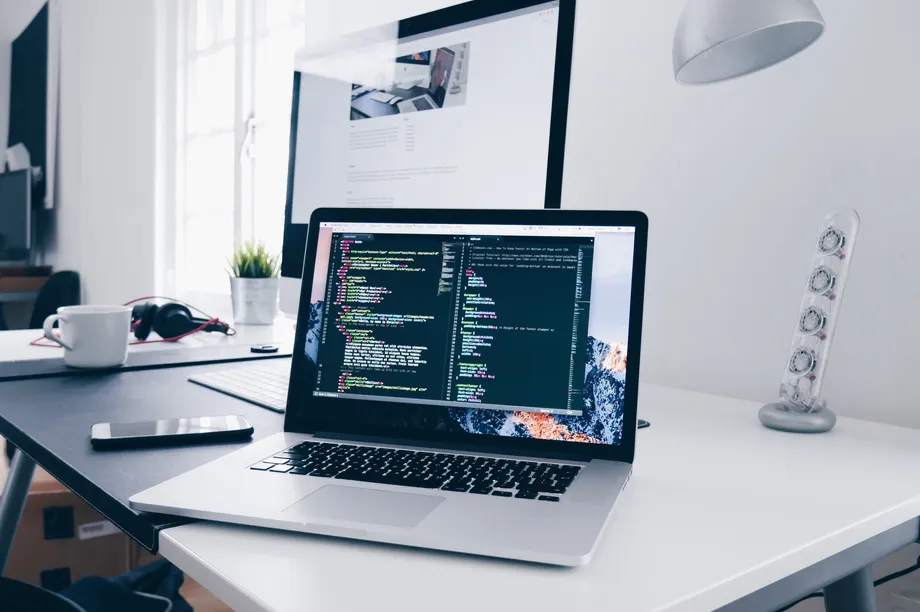
- Harsh Maur
- April 4, 2022
- 05 Mins read
- Scraping
An Ultimate Guide to Web Scraping for Beginners
Introduction
Welcome to the ultimate guide to web scraping for beginners! In the modern era where data is the new oil, understanding how to collect this data from the web is an invaluable skill. This guide aims to be your comprehensive resource for web scraping, whether you’re a beginner or looking to refine your skills. We’ll cover everything from the basics to advanced techniques, along with code examples to give you a hands-on understanding.
Table of Contents
Open Table of Contents
What is Web Scraping?
Web scraping is the automated process of extracting data from websites. Unlike manually copying and pasting information, web scraping automates this process, making it quicker and more accurate.
Basic Web Scraping Example in Python
Here’s a simple Python code snippet using the Beautiful Soup library to scrape the title of a webpage:
from bs4 import BeautifulSoup
import requests
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
title = soup.title.string
print(f'The title of the webpage is: {title}')
This code sends an HTTP request to www.example.com
, receives the HTML content, and then extracts the title tag content.
Why Web Scraping?
Web scraping serves various purposes:
-
Data Availability: The internet is a treasure trove of freely available data. Web scraping allows you to tap into this resource for various needs such as market research, sentiment analysis, and more.
-
Competitive Analysis: Businesses can scrape data from competitors’ websites to gain insights into pricing strategies, customer reviews, and product offerings.
-
Academic Research: In academia, web scraping can be used to collect data sets for research projects.
-
SEO: Web scraping can aid in SEO by collecting data on keyword rankings, backlink profiles, and competitor analysis.
How Does Web Scraping Work?
Understanding the mechanics of web scraping is crucial for effective data collection. The process can be broken down into three primary steps:
-
Sending HTTP Requests: The web scraper sends an HTTP request to the URL of the webpage you want to access. The server responds to the request by returning the HTML content of the webpage.
-
Parsing HTML: After receiving the HTML content, the web scraper parses it to extract the data needed. This is where libraries like Beautiful Soup or tools like Selenium come into play.
-
Storing Data: Once the data is extracted, it’s stored in a structured format like a CSV, JSON, or a database for further analysis or reporting.
Example: Scraping a Table
Let’s consider a Python example where we scrape a table from a webpage using the pandas
library:
import pandas as pd
import requests
url = 'https://www.example.com/table'
html = requests.get(url).content
df_list = pd.read_html(html)
df = df_list[-1]
print(df)
This code snippet fetches a table from a webpage and converts it into a pandas DataFrame, making it easier to manipulate and analyze the data.
Tools for Web Scraping
There are numerous tools and libraries available for web scraping, and choosing the right one depends on your project requirements. Here are some commonly used tools:
-
Beautiful Soup: A Python library excellent for scraping static web pages.
-
Scrapy: A more advanced Python library that offers additional features like handling cookies, user-agent strings, and more.
-
Selenium: Useful for scraping dynamic websites where the data is loaded using JavaScript.
-
Octoparse: A no-code platform for web scraping, suitable for people without a programming background.
-
WebScraper.io: A browser extension that allows you to scrape data directly from your browser.
Example: Using Scrapy
Here’s a basic example of using Scrapy to scrape quotes from a website:
import scrapy
class QuotesSpider(scrapy.Spider):
name = 'quotes'
start_urls = ['http://quotes.toscrape.com/']
def parse(self, response):
for quote in response.css('div.quote'):
yield {
'text': quote.css('span.text::text').get(),
'author': quote.css('span small::text').get(),
}
This Scrapy spider navigates to http://quotes.toscrape.com/
and extracts quotes along with their authors.
Web Scraping Techniques
Different websites have different structures and complexities, which means that the web scraping technique you choose must be suited to the specific challenges posed by each site. Here are some common techniques:
-
Static Scraping: Ideal for websites where the data is readily available in the page’s HTML. Libraries like Beautiful Soup are perfect for this.
-
Dynamic Scraping: For websites that load data dynamically using JavaScript, tools like Selenium can simulate user interactions to scrape the data.
-
API Scraping: Some websites offer APIs that provide data in a structured format. This is often the most efficient and ethical way to collect data.
-
Regular Expressions: For more complex scenarios, regular expressions can be used to extract specific patterns from the web page.
Example: Dynamic Scraping with Selenium
Here’s how you can scrape dynamic content using Selenium:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Firefox()
driver.get("https://www.example.com/dynamic")
element = driver.find_element_by_id("some_id")
element.send_keys("some text")
element.send_keys(Keys.RETURN)
result = driver.find_element_by_css_selector("output_selector").text
print(f"The result is: {result}")
driver.close()
This code snippet uses Selenium to navigate to a dynamic webpage, interact with an input field, and then scrape the resulting output.
Challenges in Web Scraping
Web scraping is powerful but not without its challenges:
-
Rate Limiting: Websites may limit the number of requests from a single IP address to prevent abuse.
-
CAPTCHAs: Some websites use CAPTCHAs to distinguish between human users and bots.
-
Legal Risks: Always make sure to read and understand a website’s terms of service before scraping. Unauthorized scraping can lead to legal consequences.
-
Data Quality: The data collected may require further cleaning and transformation to be useful.
Example: Handling Rate Limiting in Python
Here’s a Python example that handles rate limiting using the time
library:
import requests
import time
def scrape_with_rate_limiting(urls):
for url in urls:
response = requests.get(url)
process_response(response)
time.sleep(5) # Wait for 5 seconds before the next request
def process_response(response):
# Your scraping logic here
pass
This function scrapes a list of URLs while waiting for 5 seconds between each request to avoid rate limiting.
Advanced Topics
As you become more proficient in web scraping, you may want to explore some advanced topics to take your skills to the next level:
-
Web Scraping with Cloud Services: Learn how to scale your web scraping projects by leveraging cloud services like AWS Lambda.
-
Machine Learning and Web Scraping: Discover how machine learning algorithms can be applied to the data collected through web scraping for predictive analytics.
-
Real-time Web Scraping: Understand the tools and techniques for scraping data in real-time for applications like stock price monitoring or sports statistics.
Example: Web Scraping with AWS Lambda
Here’s a Python example that demonstrates how to use AWS Lambda for web scraping:
import json
import requests
def lambda_handler(event, context):
url = 'https://www.example.com'
response = requests.get(url)
data = response.json()
return {
'statusCode': 200,
'body': json.dumps(data)
}
This AWS Lambda function scrapes a website and returns the data as a JSON object. It can be triggered by various AWS services like S3, SNS, or API Gateway.
Ethical Considerations
Web scraping comes with its own set of ethical and legal considerations. Always respect a website’s robots.txt
file, which outlines what a web scraper can or cannot do on that site. Additionally, excessive scraping can overload a website’s server, which could lead to your IP address being blocked.
Example: Respecting robots.txt
in Python
Here’s how you can respect a website’s robots.txt
using Python’s robotparser
library:
from urllib.robotparser import RobotFileParser
rp = RobotFileParser()
rp.set_url("https://www.example.com/robots.txt")
rp.read()
can_scrape = rp.can_fetch("*", "https://www.example.com/some-page")
if can_scrape:
# Proceed with scraping
else:
print("Scraping is disallowed.")
This code snippet checks the robots.txt
file of a website to see if scraping is allowed for a particular URL.
Conclusion
Web scraping is an invaluable skill in the modern data-driven world. From business analytics to academic research, it offers a range of applications that are only limited by your imagination. This guide aims to be your one-stop resource for getting started with web scraping, covering everything from basic concepts to advanced techniques and ethical considerations.
By now, you should have a solid understanding of what web scraping is, why it’s important, and how to get started. So go ahead, roll up your sleeves, and dive into the world of web scraping!